API Documentation
Build custom solutions with our powerful ticketing API
Powerful, Flexible, and Secure
Our comprehensive API allows you to integrate Tixket's ticketing capabilities into your own applications, websites, and systems. Create custom experiences while leveraging our secure infrastructure.
RESTful Design
Clean, intuitive endpoints following REST principles
Comprehensive Data
Access to events, tickets, attendees, and analytics
Secure Access
OAuth 2.0 authentication and role-based permissions
High Performance
Fast response times with rate limits to ensure stability
// Example API request to create an event fetch('https://api.tixket.xyz/v1/events', { method: 'POST', headers: { 'Authorization': 'Bearer YOUR_API_KEY', 'Content-Type': 'application/json' }, body: JSON.stringify({ name: 'Summer Music Festival', start_date: '2025-07-15T18:00:00Z', end_date: '2025-07-15T23:00:00Z', venue_id: 'ven_12345', ticket_types: [ { name: 'General Admission', price: 4999, quantity: 1000 }, { name: 'VIP', price: 9999, quantity: 200 } ] }) }).then(res => res.json()).then(data => console.log(data));
API Features & Capabilities
Event Management
Create, update, and manage events programmatically...
Example Endpoints:
- GET /events
- POST /events
- GET /events/{id}
- PUT /events/{id}
- DELETE /events/{id}
Ticket Operations
Issue tickets, validate entries, and manage capacity...
Example Endpoints:
- GET /events/{id}/tickets
- POST /events/{id}/tickets
- GET /tickets/{id}
- PUT /tickets/{id}/status
- POST /tickets/{id}/validate
Attendee Management
Access attendee information while respecting privacy...
Example Endpoints:
- GET /events/{id}/attendees
- GET /attendees/{id}
- PUT /attendees/{id}/check-in
- GET /attendees/{id}/tickets
Analytics & Reporting
Access comprehensive analytics...
Example Endpoints:
- GET /events/{id}/analytics
- GET /events/{id}/sales
- GET /analytics/revenue
- GET /analytics/attendance
Webhooks
Receive real-time notifications about important events...
Example Endpoints:
- GET /webhooks
- POST /webhooks
- GET /webhooks/{id}
- PUT /webhooks/{id}
- DELETE /webhooks/{id}
User Authentication
Authenticate users securely using our zkID technology.
Example Endpoints:
- POST /auth/token
- POST /auth/verify
- POST /auth/refresh
- POST /auth/revoke
Everything You Need to Get Started
Our detailed documentation includes guides, tutorials, and reference materials to help you integrate with our API quickly and efficiently.
- Step-by-step integration guides
- Interactive API reference
- Code examples in multiple languages
- SDKs for popular platforms
- Troubleshooting and best practices
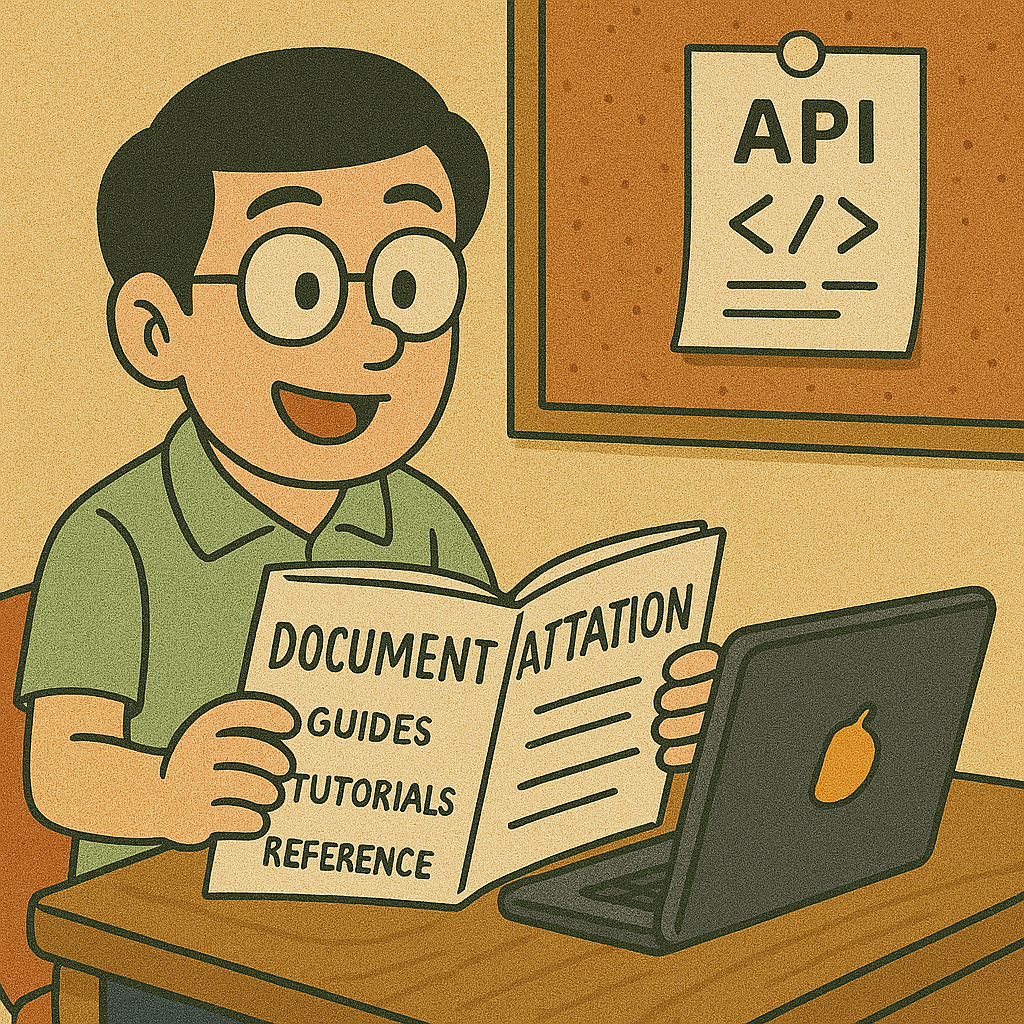